Command line arguments are the foundation of dynamic and flexible Bash scripting. By passing arguments directly to a script when executing it, users can provide different inputs without modifying the script itself. This approach increases efficiency, automates repetitive tasks, and makes scripts more reusable.
Contents
What Are Command Line Arguments?
Command line arguments are values passed to a script at execution time. These arguments can be accessed within the script through special variables:
- $0 – The name of the script itself.
- $1, $2, $3, … – Positional parameters representing individual arguments.
- $# – The total number of arguments passed.
- $@ – All arguments as separate words.
- $* – All arguments as a single string.
- $_ – The last argument of the previous command.
Understanding these special variables allows developers to create more powerful and interactive scripts.
Basic Usage of Command Line Arguments
To demonstrate how command line arguments work, consider the following simple Bash script:
#!/bin/bash
echo "Script name: $0"
echo "First argument: $1"
echo "Second argument: $2"
echo "Number of arguments: $#"
If this script is saved as script.sh and executed as follows:
bash script.sh hello world
The output will be:
Script name: script.sh
First argument: hello
Second argument: world
Number of arguments: 2
Checking for Required Arguments
It is a good practice to ensure the correct number of arguments has been passed before executing further logic. This can be done using an if
statement:
#!/bin/bash
if [ $# -lt 2 ]; then
echo "Usage: $0 arg1 arg2"
exit 1
fi
echo "Arguments received successfully."
If fewer than two arguments are provided, the script will print a usage message and exit. Otherwise, it will proceed with execution.
Looping Through All Arguments
When a script requires handling multiple arguments, a loop can be used:
#!/bin/bash
for arg in "$@"; do
echo "Processing argument: $arg"
done
This iterates through each argument separately, displaying it on the console.
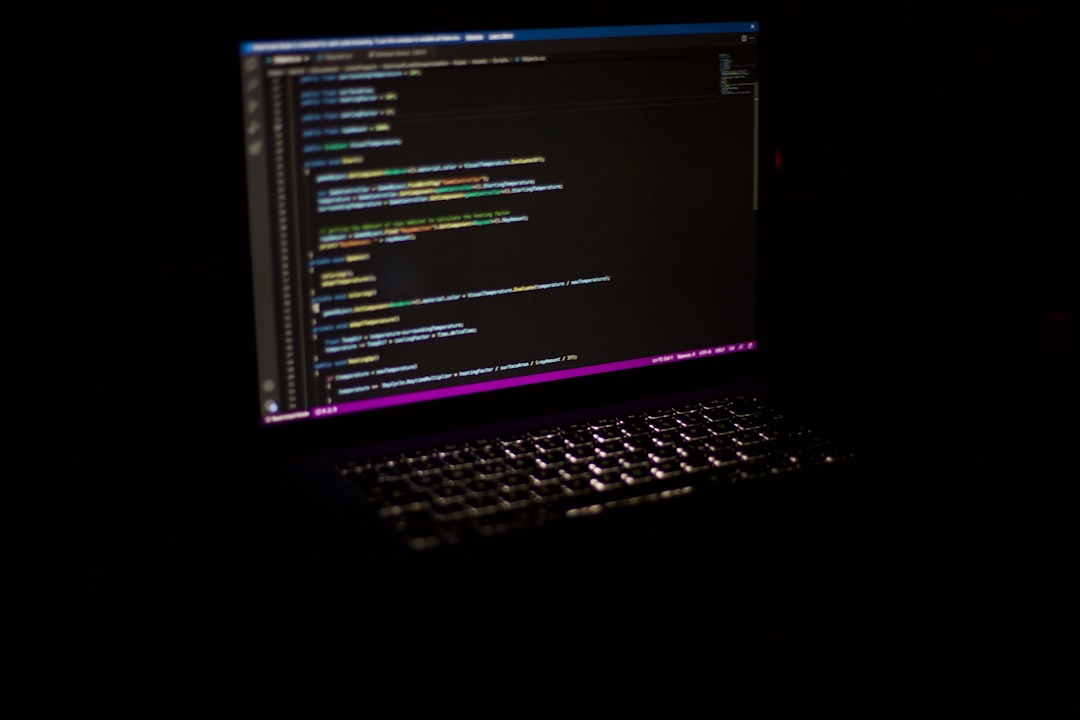
Using Command Line Arguments in Practical Scripts
Let’s consider a script that renames a file using arguments provided by the user:
#!/bin/bash
if [ $# -ne 2 ]; then
echo "Usage: $0 old_filename new_filename"
exit 1
fi
mv "$1" "$2"
echo "File renamed from $1 to $2"
Executing the script as:
bash rename.sh oldfile.txt newfile.txt
Will rename oldfile.txt to newfile.txt.
Advanced Argument Handling with getopts
For scripts that require multiple options, getopts
is useful. Consider the following example:
#!/bin/bash
while getopts "n:a:" opt; do
case $opt in
n) name=$OPTARG ;;
a) age=$OPTARG ;;
*) echo "Invalid option"; exit 1 ;;
esac
done
echo "Name: $name, Age: $age"
This script processes options -n
for a name and -a
for an age:
bash script.sh -n John -a 25
Outputs:
Name: John, Age: 25
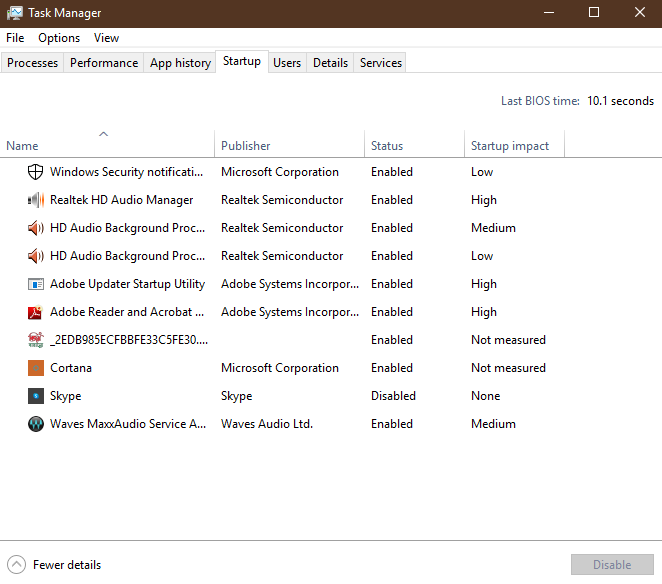
Best Practices for Command Line Arguments
- Validate Arguments: Always check if the required parameters are provided before processing.
- Provide Clear Usage Instructions: Give users an output message explaining the expected arguments.
- Use Quotes When Handling Variables: This prevents issues with spaces in arguments.
- Use getopts for Complex Input: When dealing with multiple optional parameters,
getopts
ensures efficient handling. - Exit with Meaningful Return Codes: Use
exit 1
for errors andexit 0
for success.
Conclusion
Command line arguments make Bash scripts more dynamic and useful by allowing users to interact with scripts without modifying their code. By mastering simple argument handling, validation, and using tools like getopts
, you can create powerful shell scripts that enhance automation and productivity. Implement these best practices to ensure your scripts remain robust and maintainable.